As we all know we can use roll-up summary field logic only for the master-detail relationship, but in case you have the business requirement to implement the same logic for lookup relationship then you have to think of an alternative way to cater to the requirement, there can be multiple ways to implement but in this blog, we will use a trigger to implement roll-up summary field logic. So before wasting any time, let's get started.π Read till the end to know the bonus tip.
Salesforce Developer Beginner Series
This is the salesforce CRM blog where you will learn about basics of salesforce, Triggers,Apex , integration, and lightning components: LWC and also interview questions with scenarios that will guide you to crack salesforce developer interviews.
Wednesday, 23 December 2020
Episode 13 : RollUp Summary Trigger for Lookup Relationship
Monday, 21 December 2020
Episode 12:Relationship Queries in Apex -Salesforce
In this blog, we will deep dive into the concept of relationship queries. So Before wasting any time, let's let started.π
Most of the time we need to query the data from more than one standard or custom object at a time i.e we need to traverse either from parent to child or from child to parent objects. SOQL (Salesforce Object Query Language) provides us the syntax to write these queries which are called the Relationship Queries. We can visualize the Relationship Queries same as Joins in SQL.
Note: If you are using multiple objects in the same query to fetch the data, always remember those objects must be related to each other i.e there must be a parent -to- child or child-to-parent relationships between those objects.
Parent to Child Relationship Query
We use this query when we are referring to child object records from the parent object. Here, the main object for the query is the parent object.
Standard Object Relationship
Let's take an example of the Account and Contact object where Account is the parent of Contact Object.
In order to traverse parent-to- child or child-to-parent relationship, relationship name is given to each relationship.
Drill down on the Account Name and below screen will appears and here you can find the child relationship name which is contacts in this case.
Custom Objects Relationship
For Example, there are two custom objects one is Company__c and another one is Employee__c.
One Company can have multiple employees so they share one -to -many lookup relationships where the company is the parent object and the employee is the child object.
Now If you want to reach the employee object record from the company object, you have to write your query on the parent object (Company__c in this case)and fetch the related child records( employees).
Before writing this query we need the relationship name between those objects. Here the relationship name is employees__r.
Select Id,Name ,(select Id,Name,Company__c from Employees__r) from Company__c
Note: When you are travesing from parent to child in Custom Objects Relationship, then use
plural of the child object name( without the __c) and append an __r .
NOTE: Only 1 Level of the nested query is allowed( you can remember as 1 LEVEL DOWN)
Child to Parent Relationship Query
Before jumping directly to query we need to know something.Yes , you are right we need the relationship Name between two standard objects.
Lets again take the example of Account and Contact Object where Account is the parent of the Contact Object and the value of relationship name in contact is Account and you can traverse the relationship by specifying the parent using dot notation.
For Example,
SELECT Id, Account.Name, Account.Industry, Account.Rating FROM Contact limit 10
The above query will give below results:
Custom Objects Relationship
When you use a child-to-parent relationship, you can use dot notation with the relationship name as given below:
select id, name,company__c,company__r.name from employee__c limit 10
NOTE: You can Access up to 5 levels of parents using DOT Notation( i.e 5 LEVEL UP)
Thanks for reading my blog.Stay tuned till then keep learning and keep sharing.
Saturday, 7 November 2020
Episode 11:Sharing the Record using Apex in Salesforce
Before you Proceed,
Points To Remember:
- Objects on the detail side of the master-detail relationship do not have an associated sharing object because in a master-detail relationship the child is tightly coupled with the parent hence detail record access will be determined by the masters sharing object and the relationship's sharing setting.
- The object's Organization-wide default access level must not be set to the most permissive access level i.e Public Read/Write because in this case there is no need to provide additional access.
Share Object
- ParentId: The Id of the record being shared.
- UserOrGroupId: The Id of the user or the group with whom the objects are being shared.
- Access Level: The permission level given to the user or the group.
- RowCause: The reason why the group or the user has been granted sharing access.
Apex Sharing Reason:
- Click on SetUp
- Then go to Build->Create->Objects
- Select the Object(CompetencyCourse__c in our example) for which you want to create sharing reasons.
- Then go to Apex Sharing Reasons related list.
- Click on new, the below window will appears.
- Enter the label for the sharing reason(EmployeeCourseAccess in our example)
- Enter the Name for the sharing reason(EmployeeCourseAccess in our example)
- Finally, click on the Save button.
Sunday, 27 September 2020
Episode-10: Salesforce Security Model
The Salesforce Security model is basically classified into 3 levels:
1.Object level Security
2.Field level Security.
3.Record level Security.
Before diving into it we must also understand the organization level access to the users. As an admin, you can create the users and allow them to log in to the org from the set of IP ranges and log in hours you define for them.
By default, salesforce does not restrict the login hours or IP ranges for the user but you as an admin can always configure it in order to increase the organization's security in the following ways:
- Can set up the range of IP addresses at the company level i.e users outside the range are sent an activation code for login to the org.
- Can set up the IP range at the profile level i.e those users outside this IP range will not be able to access.
- Can define the login hours at the profile level i.e if users try to access the org outside the login hours will deny access.
Object Level Security:
Objects are like tables in the database. Object-level security is managed by the profile and the permission sets. Let's understand them in detail.
Profile:
Profile controls what the user can access in the salesforce which means it controls the accessibility of objects, fields, page layouts, record types, apps, tabs, etc, and also controls system settings.
Accessibility of objects means what users can do with the objects ie CRED permissions.
C stands for CREATE
R stands for READ
E stands for EDIT
D stands for DELETE.
Each user is mandatory associated with the profile. There is a special profile of the system administrator who has the superpower to access everything in the salesforce. They have View All and Modify All access in addition to CRED access to the objects and fields.
Permission Set:
Now the question which might come in your mind is Why do we need Permission Set if the user already has the profile? Let's try to answer this and understands the concept better.
Every user is assigned one profile that provides access to the specified functions that the type of user performs but what if some users need additional access to objects and settings and you don't want to grant that additional access to everyone else having the same profile. In that case, you can assign the permission set to that particular user who needs additional access without changing his profile.
For Example:
Let's say you create a new profile name it Custom User Profile that has read, create, and edit access on the lead object. Now you want Tom who is having the Custom User Profile also to have delete access on the lead object but you don't want all other Custom User Profile users to have delete access on leads. So it doesn't make any sense to change the profile for Tom alone. Instead, you create the permission set having delete access on the lead object and assign it to Tom who needs it.
A user can have only one profile but can have zero or multiple permission sets assigned to him.
Field-level Security
Fields are similar to columns in the tables.
Field level security determines which fields the user can see and which CRED permissions the user has on the fields. It can grant or restrict the visibility to individual fields based on the user profile.
Like Object Level Security, Field-level Security is managed by the profile and the permission sets.
Profiles give baseline access to the fields and in order to give additional access to the fields other than given by the profile, we use Permission sets.
Record level Security
Records are similar to rows that contain data in the table. Record level Security controls the visibility of the records.
Record level sharing is needed when we want to share the records to the users who do not own the records. The owner of the record is the user who has created the record and has CRED access on it.
The following are the types of Record Level Security:
1.Organization-Wide Default (also known as OWD) determine the baseline access and permissions users have to the records they don't own.
Let's see the different options available for OWD:
- Private: The most restrictive setting is private as users cannot see records they do not own.
- Public Read-Only: users can view the records that they do not own but they cannot edit them or transfer the records.
- Public Read /Write: users can view and edit the records that they do not own.
- Public Read/Write/Transfer-it allows non-owners to view, edit, and change ownership. This option only applies to leads and cases.
- Controlled by Parent: It determines the access level of the child in the master-detail relationship as it is controlled by the parent.
2.Role Hierarchy
3.Sharing Rules
Role hierarchy allows us to open up the access of records vertically up in the role hierarchy pipeline of any org. But what if you want to share the records with the peers in your team or with the members of other teams then we use sharing rules to share the records horizontally. The sharing rules are used only when OWD is set anything restrictive other than public Read /Write.
- Owner Based Sharing Rules: It allows to share the records based on roles or roles and subordinates or the public groups.
- Criteria Based Sharing Rules
4. Manual Sharing
Wednesday, 9 September 2020
Episode-9 Getter and Setter Methods in Salesforce
In this blog, we will learn about using getter and setter methods in the apex controller class. Before wasting any time lets get startedπ
Saturday, 15 August 2020
Episode -8 Frequently Asked Interview Questions on Batch Apex in Salesforce
Friday, 7 August 2020
Episode 7-Batch Apex Example in Salesforce
Wednesday, 29 July 2020
Episode 6-Asynchronous Apex-Future vs Queueable vs Batch Apex in Salesforce
Wednesday, 22 July 2020
Episode 5-The Complete Guide on Future Methods in Salesforce
Saturday, 18 July 2020
Episode 4-Conceptual Questions on Apex Triggers
Sunday, 12 July 2020
Episode 3-Salesforce Triggers and Scenario Based Questions
Trigger <TriggerName> On <ObjectName> (trigger_events) {
//code
}
- Before Insert
- Before Update
- Before Delete
- After Insert
- After Update
- After Delete
- After UnDelete
Context Variables: In order to access the records which are fired due to the Trigger, We use Context Variables in Trigger. For Example, the most commonly used Context Variables are Trigger.New and Trigger.Old.
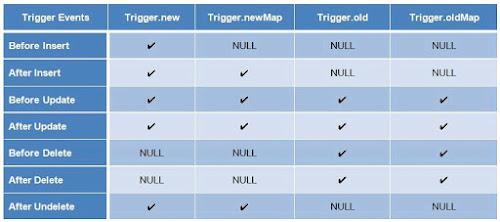
Implementing the Trigger Scenarios:
Saturday, 4 July 2020
Episode 2-Ten Most Important Topics you need to prepare for Salesforce PD1 Certification
Saturday, 27 June 2020
Episode 1- Ten Most Frequently Asked Questions for Salesforce Developer Interview
Episode 13 : RollUp Summary Trigger for Lookup Relationship
As we all know we can use roll-up summary field logic only for the master-detail relationship, but in case you have the business requiremen...

-
Security is always crucial to any organization which cannot be compromised at any level. Therefore understanding the security model in sal...
-
As we all know we can use roll-up summary field logic only for the master-detail relationship, but in case you have the business requiremen...
-
If you are preparing for a Salesforce interview and you want one stop blog where you can brush up your knowledge in very less time ,you a...